The errors that occur during the execution of a Python program are called exceptions. Examples of exceptions include dividing by zero, combining objects of incompatible types, and many others. Some exceptions have specific names, such as ZeroDivisionError and TypeError. If exceptions are not handled properly, they can halt the entire execution of the program. This is sometimes necessary, for instance if there is an issue with a variable that has not been defined. However, it may not always be desirable, as certain exceptions, such as division by zero, may be caused by missing data rather than a significant issue with the program.
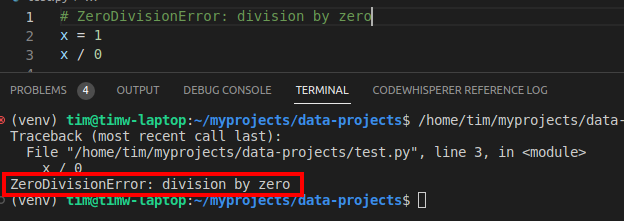
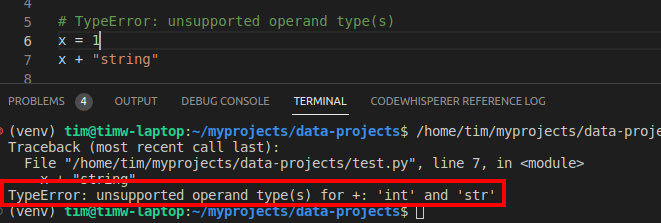
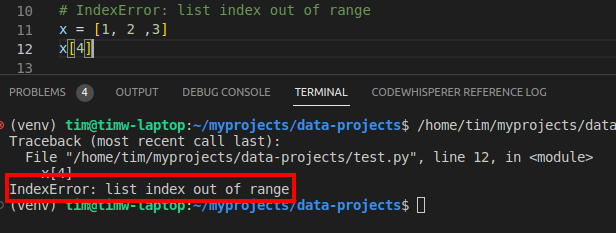
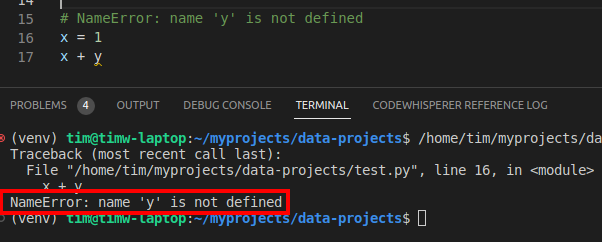
try-except-finally
To handle exceptions in Python, you can use the try-except-finally code structure. The code that may potentially cause an exception is placed in a “try” block, followed by an “except” block containing the name of the specific exception to be handled and the code to be executed if that exception is raised. You can also have multiple “except” blocks to handle different types of exceptions. The optional “finally” block contains code that will be executed no matter what, and is often used for cleanup tasks such as closing opened files. If an exception is raised and handled, the program will not terminate but will instead execute the code in the corresponding “except” block.
# Example syntax
try:
# Try running some code here
except ExceptionNameHere:
# Run this code if ExceptionNameHere happens
except AnotherExceptionNameHere: # <- multiple exception blocks accepted
# Run if AnotherExceptionNameHere happens
finally: # <- optional
# Run this code no matter what
Raising exceptions
It is possible to intentionally raise exceptions in your code using the “raise” keyword, which can be followed by a custom error message in parentheses. This allows you to specify specific conditions that must be met and to communicate any deviations from those conditions to the user of the code, who can choose to handle the exception using a try-except block.
# Example syntax
raise ExceptionNameHere('Error message here')
Exceptions in Python can be a useful tool for handling errors and exceptional cases in your code. This is just the tip of the iceberg when it comes to working with exceptions. I encourage you to experiment and learn more about this topic.
Tim